Vue.js for React devs: Who Wins the Burger Building Contest?
React has been one of the most popular JavaScript libraries for building user interfaces in recent years. However, if you’re a React developer, you might be curious about trying out Vue.js. In this blog post, we will explore the similarities and differences between React and Vue.js and how you can leverage your React knowledge to learn Vue.js. Using simple examples, we will compare concepts of React with their implementation in Vue.js. Building components in React and Vue.js is like creating burgers with the same ingredients combined in different ways. In the following examples we will create our own burger components by comparing different approaches and syntax. So, let’s see which one is more fun to cook and which one tastes better in the end.
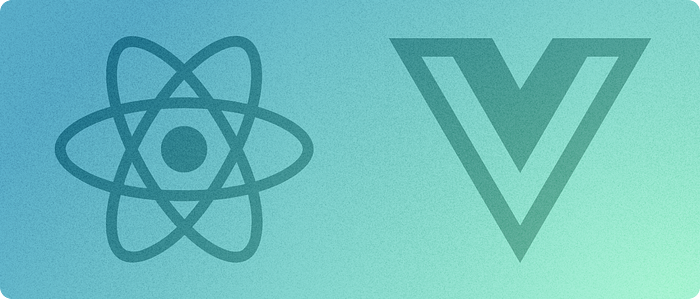
Similarities
React and Vue.js share some similarities. Both are JavaScript libraries that allow us to create web user interfaces. Both of them use a virtual DOM, which allows for efficient updates to the UI without having to re-render the entire page. Both also have a component-based architecture, meaning you can break down your UI into smaller, reusable components. In addition, both React and Vue.js offer great developer tools, such as React Developer Tools and Vue.js Devtools, respectively. Both libraries are open-source and promise to be fast and lightweight.
Differences
One key difference between React and Vue.js is that while React is primarily a library for building user interfaces, Vue.js aims to be a fully-fledged framework. This means that Vue.js comes with more out-of-the-box features and tools that enable developers to build complex applications with less configuration and setup.
Another difference is the syntax on how to render content to a page. While we use JSX to create components in React, Vue.js uses HTML templates (with the option to write JSX). One benefit of Vue’s separation of HTML, CSS and JS is the flatter learning curve for developers who are familiar with the traditional approach of writing HTML, CSS, and JavaScript.
Of course, as a React developer, you want to know the differences between React and Vue.js when creating components and interfaces, so let’s dive into the differences in syntax and usage between the two libraries. In this article I will compare React functional components with the Vue.js options API. We will come back to Vue’s compositions API in a future blog post, but for an overall comparison, the options API is easier to start with.
Component Structure
Let’s see how writing components differ between React and Vue.js. For that, we will use a simple example: let’s say we want to create a burger, which is one component that consists of different sub-components. A functional component in React could look something like this:
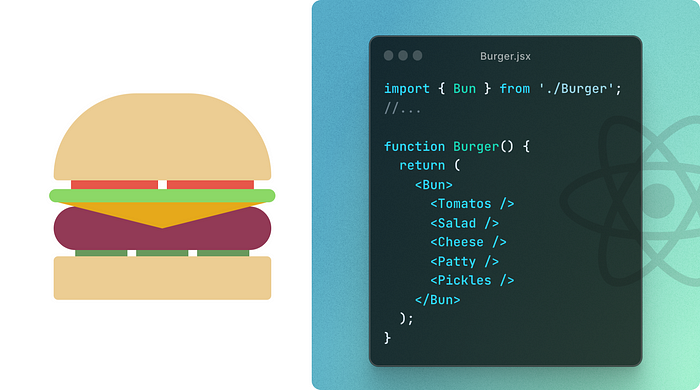
In Vue.js, a similar component could be created using a single-file component that includes a template, script, and style section, where the template section contains the HTML structure of the component, and the script section contains the component’s logic and exposes the component as the file’s default export. To use other components as child components, they must be imported and registered with the components
option.
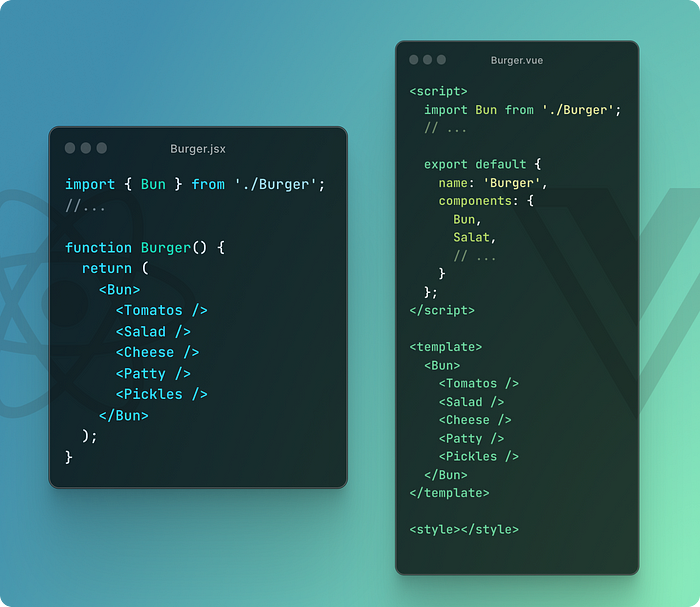
Props
We use props to pass information to child components and let components communicate with each other. In React, we read the props a component receives from the parameters of the functional component. To pass them to the child component, we add them to the JSX tag.
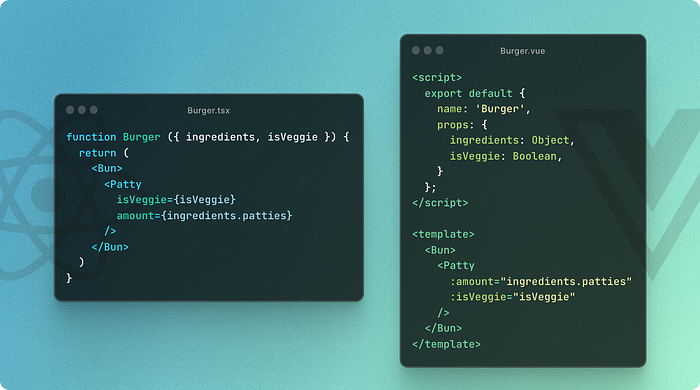
To pass props to child components in Vue.js, we have to use different “directives” that define certain forms of behavior for the component itself and its props. To bind the value of a prop to the component in one way, we need to use the v-bind
directive as a prefix, or its shorthand :
. Similar to the curly braces in React, we use this directive for any prop that is not a string.
To read the props a component receives, we must define each prop inside the props
option.
Conditional Rendering
Often, we want to display certain elements depending on different conditions. To render JSX based on these conditions, we can use operators like ?
and &&
or use if
statements in React.
To conditionally render elements, Vue.js provides different directives such as v-if
/ v-else
(where the element is not rendered) or v-show
(which renders, but hides the element).
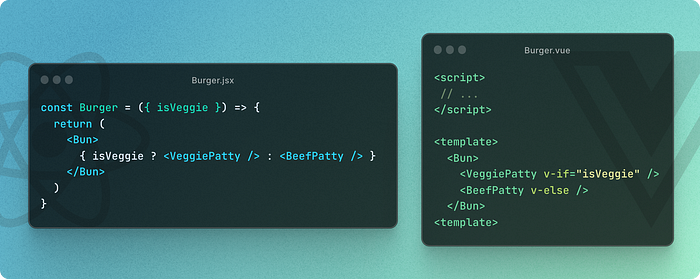
List Rendering
If we want to render a component multiple times based on a collection of data. In React, we use JavaScript Array methods such as map
that transform our data collection into a list of components.
As Vue.js provides another directive to achieve this goal. We can simply use the v-for
directive on a component that should be rendered as list element and pass an array to this prop.
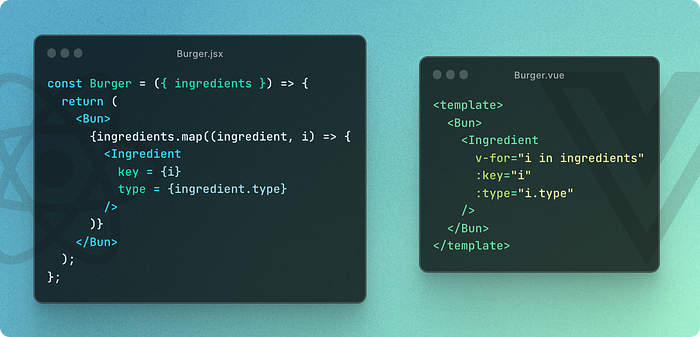
Component State and Methods
To manage the state of a component, we primarily use the useState
hook in React. All the logic of a component is directly defined inside the component function itself.
In our example, we want to be able to modify the number of each ingredient in our burger. For that, we create a Builder that lets us select our preferred ingredients.
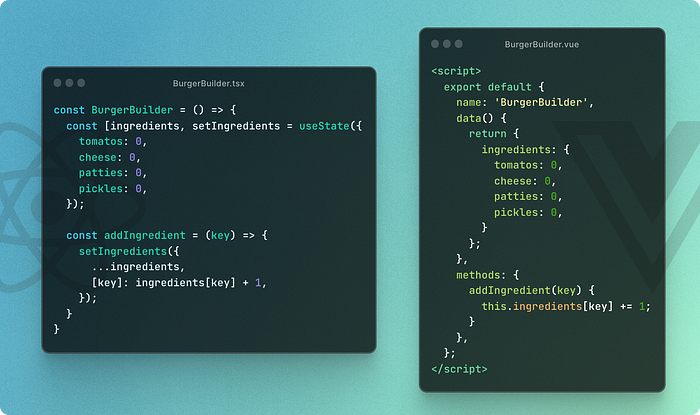
In Vue.js, the process of defining and modifying the state is quite simple, compared to React’s approach. The data
option initializes the state and exposes each member, which can be directly updated without the need for a setter function.
Component functions are defined inside the methods
option. Members of a component need to be accessed via this
.
Events
If we want to pass an event handler to a component in React, we first define a function that we pass as a prop to the respective JSX tag. We can simply pass this prop down the component tree and call the handler in the component that emits the event.
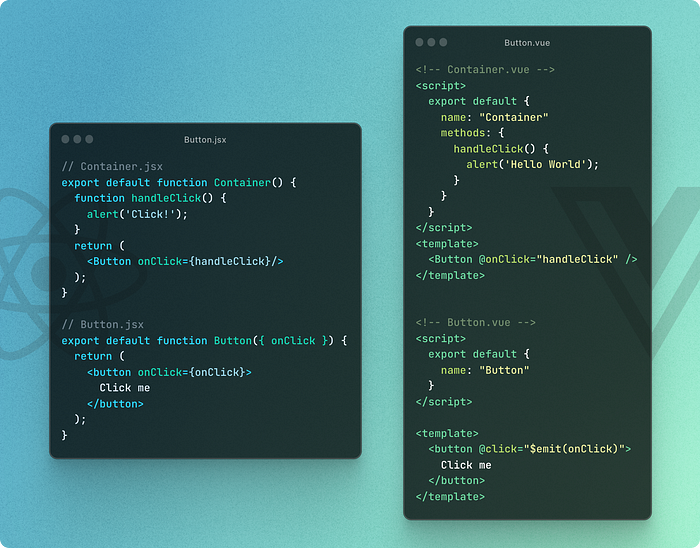
Passing event handlers in Vue.js works differently in some ways. To bind a handler to its event, we use the v-on
directive (@
as shorthand). This handler is not passed down the component tree to the respective child. In order to bubble the event up the tree, each component has to emit a new event, and its parent can listen to it via the v-on
directive.
Lifecycle hooks
Every React component goes through the same basic lifecycle — mount, update, and unmount. While we might use methods like componentDidMount
in class components, we use the useEffect
hook in functional components to respond to changes in props or state.
While the useEffect
hook provides more flexibility, as it can be used to handle various actions, Vue.js provides more explicit hooks for handling actions in a component’s lifecycle. We are able to use methods such as created
, beforeMount
, updated
, or beforeUnmount
. These methods offer a simple way to perform actions throughout the component’s lifecycle, and especially for beginners, they might be easier to understand than the proper usage of the useEffect
hook in React.
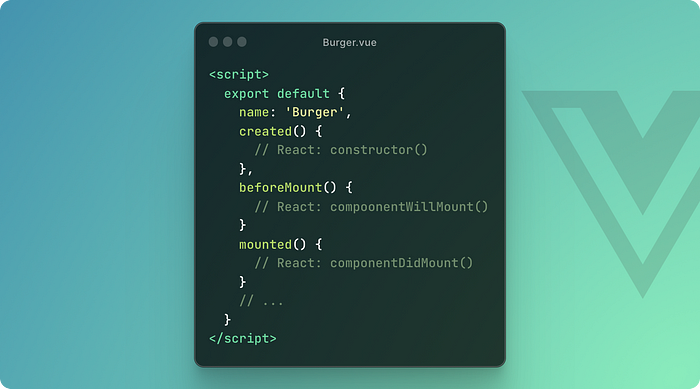
Watchers and Observables
In addition to lifecycle methods, Vue.js also offers an explicit concept to register to changes of a specific state. Where we might also use the useEffect
hook in React, that is, for example, called whenever a state changes, we can implement the watch
method inside a Vue component. The name of a watcher function always reflects the variable it depends on.
In some cases, we want to derive some value from the current value of a state in our component. Where we would use the useMemo
hook in React, Vue.js provides “computed properties”, that update dynamically whenever the value of a dependent state changes. Here, the dependencies do not have to be specified explicitly, like in React, Vue.js handles them implicitly.
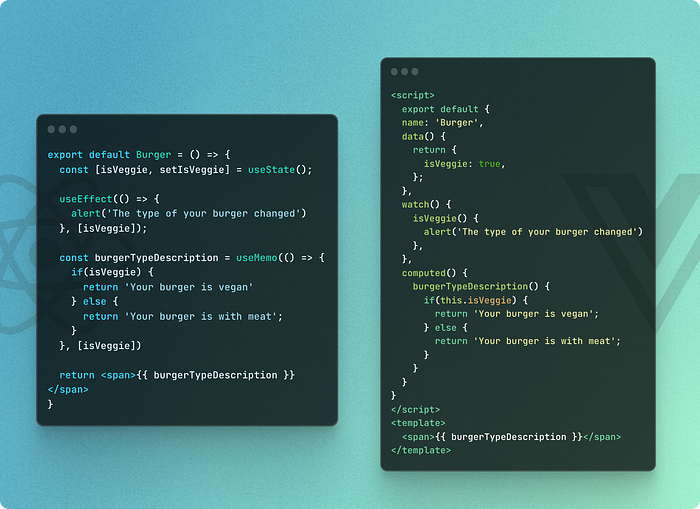
Passing Child Elements
In many cases we want to nest components or content into other components. In React, we would nest this content inside the JSX tag of our component, which receives this content as children
prop which can then be rendered by the component.
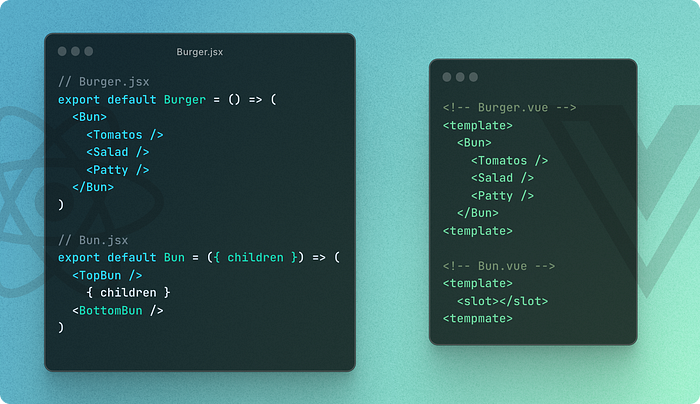
In Vue.js we also simply nest the content into the tags of our component. However, inside the component, we have to define a slot
which determines where the child content should be rendered. In contrast to React, slots are more versatile and for example, allow us to define named slots as distinct placeholders for content that can be filled by the parent component.
Conclusion
In this article, I gave a quick overview of some similarities and differences between React and Vue.js to give you, as a React developer, an idea about how writing user interfaces in Vue.js can feel. Both libraries are great for building fast and lightweight interfaces, and none are superior to another. Choosing the right framework and libraries always depends on what you need and which style you prefer.
After working on projects both implemented with React and Vue.js, they both have their pros and cons. While Vue.js is easier to learn for people who are already familiar with HTML and CSS, the concept around HTML templates sometimes leads to more boilerplate and less comprehensible code. Especially in large applications, I was faced with the challenge to find ground in a large code base. React, on the other hand, has a steeper learning curve, but for me, it provides a more lightweight approach to build reusable components that scale well with a growing product.
In the end, everyone has their own unique taste in how they like to put together their burgers with the ingredients they prefer. With React, I like the complete control over every ingredient and the flexibility of the JavaScript approach combined with a well-established and documented ecosystem. But I also like Vue’s simplicity and ease of use, especially for smaller projects or for developers who are new to building web applications. Reactivity and data binding in Vue.js are straightforward and easy to implement, compared to hooks in React which are sometimes more complex and require a deeper understanding of JavaScript concepts.
Nevertheless, both libraries are great for building web interfaces, and provide rich ecosystems and active communities.
It’s time to build
At & we see the strengths and capabilities of both Vue.js and React. We understand that projects have different requirements and preferences. We choose the frameworks and libraries that fit best to the project, the client and the team to build web applications that are robust and scalable.
Let me know about your opinion and feel free to reach out if you have any questions.